测试开发技术网站
博客
设计
设计
开发
Python
测试
unittest
运维
运维
CI/CD
CI/CD
数据库
数据库
云计算
云计算
云原生
云原生
爬虫
爬虫
数据分析
数据分析
人工智能
人工智能
登录
注册
Pytest----Pytest快速体验
收藏本文
作者:redrose2100 类别: 日期:2022-09-01 07:47:32 阅读:564 次 消耗积分:0 分
[TOC] 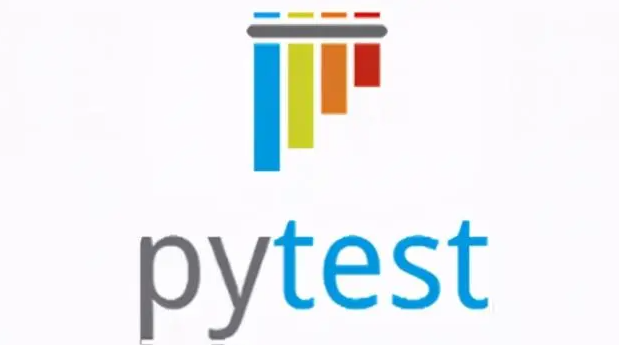 # 一、Pytest简介 Pytest是一款基于python开源的自动化测试框架,具体来说它有以下特点: * 使用简单,非常容易上手 * 功能强大,几乎可所有测试活动的自动化 * 开源生态健康,文档丰富 * 插件化架构,可扩展性强 * 拥有非常丰富的插件库,几乎可覆盖现有的大部分自动化需求 * 支持多种执行方式,执行调度灵活 * 支持参数化,支持数据驱动测试 * 能够兼容其他自动化测试框架编写的脚本 * 结合allure插件生成非常漂亮的测试报告 * 方便地与jenkins进行持续集成 # 二、Pytest的安装与升级 (1) 安装 ```bash pip install pytest ``` (2)查看版本,如下表示安装OK ```bash $ pytest --version pytest 7.1.2 ``` (3)升级 ```bash pip install -U pytest ``` # 三、创建并执行第一个测试脚本 (1)四行代码即可写一个测试脚本,如下,文件命名为 test_sample.py ```python def func(x): return x + 1 def test_answer(): assert func(3) == 5 ``` (2)直接使用pytest命令即可执行 ```bash $ pytest ========================================================================= test session starts ========================================================================== platform win32 -- Python 3.9.13, pytest-7.1.2, pluggy-1.0.0 rootdir: D:\redrose2100-book\ebooks\Pytest企业级应用实战\src collected 1 item test_demo.py F [100%] =============================================================================== FAILURES =============================================================================== _____________________________________________________________________________ test_answer ______________________________________________________________________________ def test_answer(): > assert func(3) == 5 E assert 4 == 5 E + where 4 = func(3) test_demo.py:5: AssertionError ======================================================================= short test summary info ======================================================================== FAILED test_demo.py::test_answer - assert 4 == 5 ========================================================================== 1 failed in 0.13s =========================================================================== ``` 执行结果中100%表示测试脚本执行进度,即所有用例均已执行完成,此外在执行结果最后,pytest打印出了执行测试报告,即有一个用例失败,func(3)的值为4,与期望值5不相等。 断言这里使用了assert语句,关于assert断言语句的高级应用在后续文章中继续深入讲解,这里仅仅体验一下。 # 四、pytest命令的默认行为 pytest命令这里暂时知道会执行当前目录以及当前目录的所有子目录中的test_*.py 文件和 *_test.py 文件中的测试脚本,关于更多规则细节也将在后续篇章中详细展开讲解。 # 五、对产生的异常进行断言 通过raise可以对代码产生的异常进行断言,如下代码 ```bash import pytest def f(): raise SystemExit(1) def test_mytest(): with pytest.raises(SystemExit): f() ``` 执行pytest命令,结果如下: ```bash pytest ========================================================================= test session starts ========================================================================== platform win32 -- Python 3.9.13, pytest-7.1.2, pluggy-1.0.0 rootdir: D:\redrose2100-book\ebooks\Pytest企业级应用实战\src collected 1 item test_demo.py . [100%] ========================================================================== 1 passed in 2.03s =========================================================================== ``` 关于断言的更多的内容,将在后续篇章中详细展开讲解。 # 六、在类中编写测试脚本 当编写多个测试脚本时,可以考虑使用类将他们组织起来,这样便于管理。如下创建测试文件test_demo.py,并编写类TestClass,注意类名不要Test开头,此外,类中不能有_init__(self) 方法。如下,测试类TestClass2中有__init__(self)方法。 ```python class TestClass: def test_one(self): x = "this" assert "h" in x def test_two(self): x = "hello" assert hasattr(x, "check") class TestClass2: def __init__(self): pass def test_one(self): x = "this" assert "h" in x def test_two(self): x = "hello" assert hasattr(x, "check") ``` 然后使用pytest命令执行,结果如下: ```bash $ pytest ========================================================================= test session starts ========================================================================== platform win32 -- Python 3.9.13, pytest-7.1.2, pluggy-1.0.0 rootdir: D:\redrose2100-book\ebooks\Pytest企业级应用实战\src collected 2 items test_demo.py .F [100%] =============================================================================== FAILURES =============================================================================== __________________________________________________________________________ TestClass.test_two __________________________________________________________________________ test_demo.py:10 D:\redrose2100-book\ebooks\Pytest企业级应用实战\src\test_demo.py:10: PytestCollectionWarning: cannot collect test class 'TestClass2' because it has a __init__ construc tor (from: test_demo.py) class TestClass2: -- Docs: https://docs.pytest.org/en/stable/how-to/capture-warnings.html ======================================================================= short test summary info ======================================================================== FAILED test_demo.py::TestClass::test_two - AssertionError: assert False ================================================================ 1 failed, 1 passed, 1 warning in 0.08s ================================================================ ``` 可以发现这里有一个用例通过,一个失败,其中还有一个告警,"cannot collect test class 'TestClass2' because it has a __init__ construc tor"即TestClass2中无法采集用例,因为它有__init__构造方法。 使用类组织测试函数有许多好处,如: * 可以有效的组织用例 * 可以仅在类中共享fixture * 可以在类上打标签从而对类中的所有用例打标签 但是在使用类组织测试函数时需要特别注意,测试类中的每个用例都是测试类的一个独立的对象,类中的用例如果通过类属性共享变量将是非常糟糕的设计,如下,value是类变量,在test_one和test_two两个测试用例中共享,但是在test_one对value进行了修改,通过后续的执行pytest命令的时候发现在test_one中的修改并未修改test_two中的value的值,因此这种很容易引起误解的设计师非常糟糕的。在自动化实践中要尽量避免这类设计。 ```python class TestClassDemoInstance: value = 0 def test_one(self): self.value = 1 assert self.value == 1 def test_two(self): assert self.value == 1 ``` 执行pytest命令,结果如下: ```bash $ pytest ========================================================================= test session starts ========================================================================== platform win32 -- Python 3.9.13, pytest-7.1.2, pluggy-1.0.0 rootdir: D:\redrose2100-book\ebooks\Pytest企业级应用实战\src self =
def test_two(self): > assert self.value == 1 E assert 0 == 1 E + where 0 =
.value test_demo.py:9: AssertionError ======================================================================= short test summary info ======================================================================== FAILED test_demo.py::TestClassDemoInstance::test_two - assert 0 == 1 ===================================================================== 1 failed, 1 passed in 2.15s ====================================================================== ``` # 七、测试脚本请求创建一个临时目录 pytest提供了许多内置的fixture,比如tmp_path,将fixture名字写入测试函数的参数位置,就会在执行测试函数之前,率先执行fixture的动作,比如tmp_path,就会在执行测试函数之前创建一个临时目录,如下代码: ```bash def test_needsfiles(tmp_path): print(tmp_path) assert 0 ``` 使用pytest -q,即输出简要信息,执行结果如下: ```bash pytest -q F [100%] =============================================================================== FAILURES =============================================================================== ___________________________________________________________________________ test_needsfiles ____________________________________________________________________________ tmp_path = WindowsPath('C:/Users/hitre/AppData/Local/Temp/pytest-of-hitre/pytest-4/test_needsfiles0') def test_needsfiles(tmp_path): print(tmp_path) > assert 0 E assert 0 test_demo.py:3: AssertionError ------------------------------------------------------------------------- Captured stdout call ------------------------------------------------------------------------- C:\Users\hitre\AppData\Local\Temp\pytest-of-hitre\pytest-4\test_needsfiles0 ======================================================================= short test summary info ======================================================================== FAILED test_demo.py::test_needsfiles - assert 0 1 failed in 0.07s ``` 可以ka看到在执行测试函数之前创建了 WindowsPath('C:/Users/hitre/AppData/Local/Temp/pytest-of-hitre/pytest-4/test_needsfiles0') 的临时目录。 这里仅演示fixture的神奇的作用,在后续篇章中将对fixture的更多的功能详细展开讲解。
始终坚持开源开放共享精神,同时感谢您的充电鼓励和支持!
版权所有,转载本站文章请注明出处:redrose2100, http://blog.redrose2100.com/article/355
上一篇:
SonarQube----SonarQube和Gitlab集成
下一篇:
Pytest----Pytest自动化脚本默认的命名规则以及如何定制自定义的命名规则
搜索
个人成就
出版书籍
《Pytest企业级应用实战》
测试开发技术全栈公众号
测试开发技术全栈公众号
DevOps技术交流微信群
加微信邀请进群
常用网站链接
开源软件洞察
云原生技术栈全景图
Python语言官方文档
Golang官方文档
Docker官方文档
Jenkins中文用户手册
Scrapy官方文档
VUE官方文档
Harbor官方文档
openQA官方文档
云原生开源社区
开源中国
Kubernetes中文文档
Markdown语法官方教程
Kubernetes中文社区
Kubersphere官方文档
BootStrap中文网站
JavaScript中文网
NumPy官方文档
Pandas官方文档
GitLink确实开源网站
数据库排名网站
编程语言排名网站
SEO综合查询网站
数学加减法练习自动生成网站
Kickstart Generator
文章分类
最新文章
最多阅读
特别推荐
×
Close
登录
注册
找回密码
登录邮箱:
登录密码:
图片验证码:
注册邮箱:
注册密码:
邮箱验证码:
发送邮件
注册邮箱:
新的密码:
邮箱验证码:
发送邮件